Table of Contents
Data Seeding
in this article, we will talk about data seeding. Data seeding is a process where you populate a database with an initial set of data.
There are several ways to accomplish this in Entity Framework Core (EF Core):
- Model seed data
- Manual migration customization
- Custom initialization logic
Seed data in migration
in this article, we will discuss the first scenario: how to seed data in migration using the “Model seed data” approach.
In EF Core, seeding data can be part of the model configuration. This way, EF Core migrations can automatically compute what insert, update, or delete operations need to be applied when upgrading the database.
For this example, in our project, we will add a new Model Category, then attach this model in the DbContext using the DbSet (Entity Set).
here is the class/Model Category :
public class Category
{
public int Id { get; set; }
public string? Name { get; set; }
}
and here are the changes we need to make to the DbContext
public class AppDbContext : DbContext
{
protected override void OnConfiguring(DbContextOptionsBuilder options)
{
options.UseSqlServer(@"Server=.\SQLEXPRESS; Database=EFCoreDb;Trusted_Connection=True;Encrypt=False;");
}
public DbSet? Books { get; set; }
public DbSet? Categories { get; set; }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity().HasData(
new Category {Id=1, Name = "Web" },
new Category {Id=2, Name = "Development" },
new Category {Id=3, Name = "Design" },
new Category {Id=4, Name = "Marketing" }
);
}
}
After these changes, we will execute the commands that allow us to create the migration for the Category model and update the database.
to generate the migration script :
dotnet ef migrations add addCategory
to update the database and craeate table categories :
dotnet ef database update
After the execution of these two commands, we will create the Categories table loaded with the following data as follows:
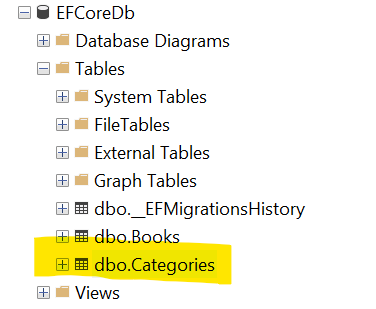
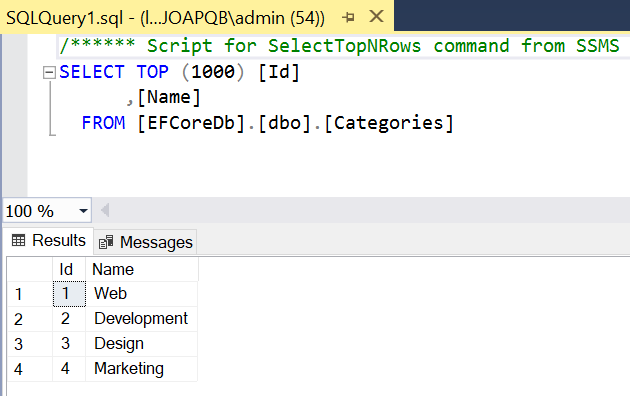
when using the “Model seed data” approach we must consider the following points:
- The primary key value must be specified, even if it is usually generated by the database. It will be used to detect data changes between migrations
- If the primary key is changed in any way, previously seeded data will be removed.