Table of Contents
Remove Migration
In many cases and after applying one or more migrations, you may find that you need to make additional changes to your EF Core model (add or remove a property, change the data type, etc.), in this case, we need to remove this migration in order to readjust the model with these new properties.
Why remove migration
In Entity Framework Core (EF Core), when your model changes, you also need to update your database tables to keep everything in sync for your application to function correctly.
so we need to remove a migration in order to make necessary changes to the structure of our database after modifying a model related to the database.
We can remove migration by executing this command :
CLI : Command-line interface
dotnet ef migrations remove
Package Manager Console
PM> remove-migration
But the above command will precisely delete the last migration executed and which is not yet applied to the database with the “Database update” command and revert the model snapshot to the previous migration.
N.B : if that migration is already applied to the database, so “remove-migration” will throw the following exception:

Reverting a Migration
If you have already applied a set of migrations to the database and want to revert to a specific migration, you can of course do so by following the steps below:
First, it’s useful to know all the migrations you have already applied, You can list all existing migrations as follows:
.NET CLI : Command-line interface
dotnet ef migrations list
Package Manager Console
PM> Get-Migration
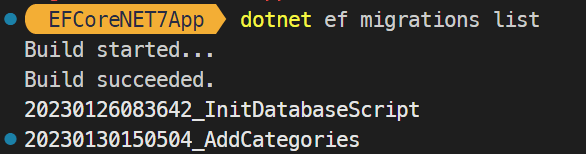
To unapply a specific migration, use the command :
- CLI : dotnet ef database update MigrationName
- PM : Update-Database -Migration MigrationName
For some reason, you want to restore the database to its previous state, for example to the state in migration “InitDatabaseScript“. In this case, we can use the following commands to revert the database to the specified previous migration snapshot.
.NET CLI : Command-line interface
dotnet ef database update InitDatabaseScript
Package Manager Console
PM> update-database InitDatabaseScript
After executing this command we will restore our database to the state and configuration required by the InitDatabaseScript migration by making the following changes:
- No changes in the script files of previously executed migrations (like “AddCategories” migration);
- Deletions of tables created by migrations that come after InitDatabaseScript;
- Updating the __EFMigrationsHistory table.
All the changes made will be illustrated in the following images :
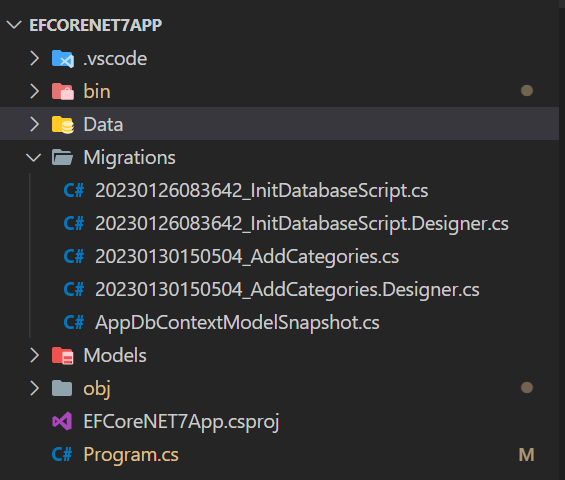
migrations files scripts : No changes in migrations scripts files
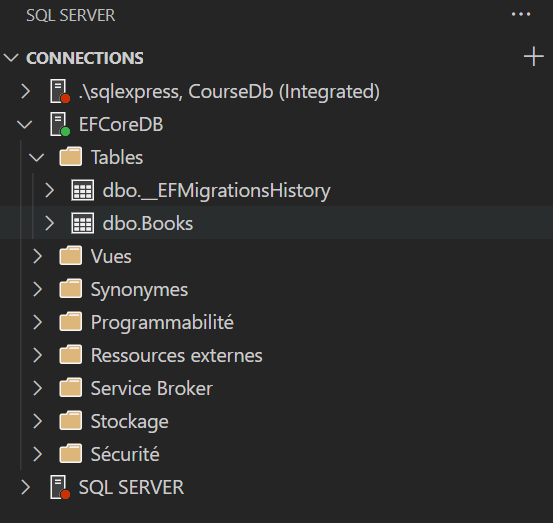
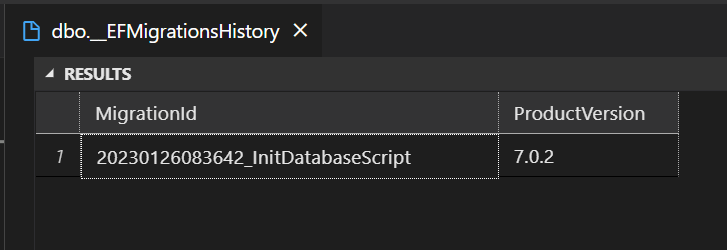
EFMigrationsHistory table : This table in your database used for storing meta data about your migrations.
If you want to revert or unapply all migrations we can use the following commands:
- CLI : dotnet ef database update 0
- PM : Update-Database -Migration 0
The number 0 is a special case that means before the first migration and causes all migrations to be reverted.
if we run this command on our project, we will initialize all migrations like this:
dotnet ef database update 0
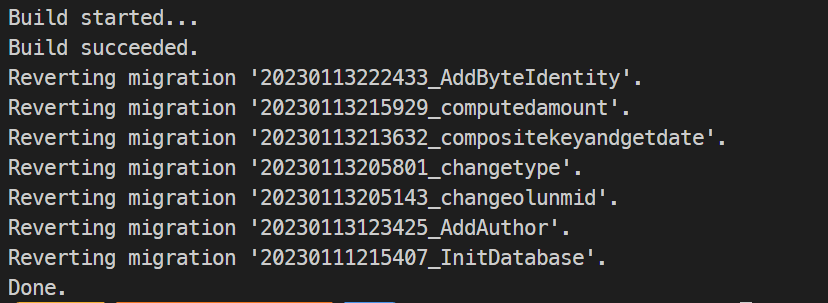
Resetting all Migrations manually
In extreme cases, it may be necessary to remove all migrations and start over. This can be easily done by deleting the migrations folder and database. At this point, you can create a new initial migration containing your current schema.
Clean migrations files without losing data
working with the code-first approach in Entity framework core is great, but migration files accumulate over time and depending on the size of the project. we will be in front of dozens of files. Sometimes you need to clean all these files and keep a single file containing all the migration scripts.
To clean these migration files we will follow six instructions below :
Step 1 : Delete your _EFMigrationsHistory table
this table stores meta data about your migrations
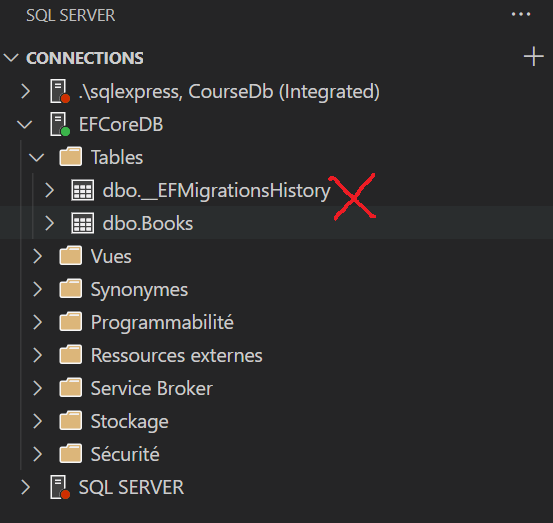
Step 2 : Delete your migration files
In solution explorer locates and deletes all migrations files
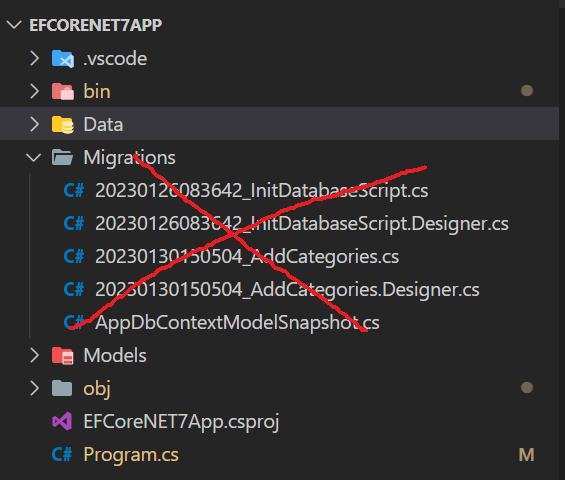
Step 3 : Generate a new migration script
To create a new migrations script file, you can use .NET CLI or the Package Manager Console to execute the following command.
- CLI : dotnet ef migrations add initial
- PM : Add-Migration initial
N.B : You can name your migration whatever you want but initial seems to fit the purpose.
after executing this command, we will generate a single file that contains all migrations named “initial.cs“.
Step 4 : Fake Update database
We now have our migration, but we like the database to stay as it is, which means no data loss
This means that we don’t actually want to run the migration. However, we need early code migrations to believe we’ve done it.
we can realize that by commenting out the code in Up() method inside our migration file. This way we can run an update database command without any SQL update being run and no changes in the database.
public partial class initial : Migration
{
protected override void Up(MigrationBuilder migrationBuilder)
{
/* migrationBuilder.CreateTable(
name: "Books",
columns: table => new
{
Id = table.Column(type: "int", nullable: false)
.Annotation("SqlServer:Identity", "1, 1"),
Name = table.Column(type: "nvarchar(max)", nullable: false),
Description = table.Column(type: "nvarchar(max)", nullable: true)
},
constraints: table =>
{
table.PrimaryKey("PK_Books", x => x.Id);
});
migrationBuilder.CreateTable(
name: "Categories",
columns: table => new
{
Id = table.Column(type: "int", nullable: false)
.Annotation("SqlServer:Identity", "1, 1"),
Name = table.Column(type: "nvarchar(max)", nullable: true)
},
constraints: table =>
{
table.PrimaryKey("PK_Categories", x => x.Id);
});
migrationBuilder.InsertData(
table: "Categories",
columns: new[] { "Id", "Name" },
values: new object[,]
{
{ 1, "Web" },
{ 2, "Development" },
{ 3, "Design" },
{ 4, "Marketing" }
});
*/
}
protected override void Down(MigrationBuilder migrationBuilder)
{
migrationBuilder.DropTable(
name: "Books");
migrationBuilder.DropTable(
name: "Categories");
}
}
Step 5 : Run Update database
After commenting out the code in Up() method, run update-database command under the package manager console or dotnet ef database update if using .NET CLI, this step will update __EFMigrationsHistory table.
CLI > dotnet ef database update
PM > update-database
Step 6 : Uncomment Up() Method
Now it’s time to Uncomment the contents of Up() method.